[ad_1]
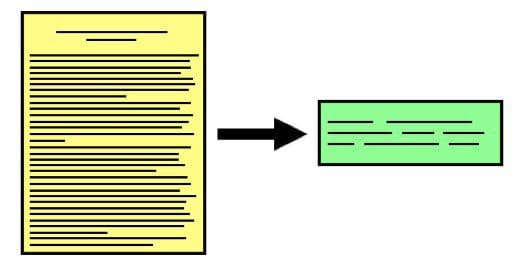
Automated text summarization refers to performing the summarization of a doc or paperwork utilizing some type of heuristics or statistical strategies. A abstract on this case is a shortened piece of textual content which precisely captures and conveys an important and related info contained within the doc or paperwork we would like summarized.
There are 2 classes of summarization strategies: extractive and abstractive. We’ll deal with the usage of extractive strategies herein, which perform by figuring out the essential sentences or excerpts from the textual content and reproducing them verbatim as a part of the abstract. No new textual content is generated; solely current textual content is used within the summarization course of. This differs from abstractive strategies, which make use of extra highly effective pure language processing strategies to interpret textual content and generate new abstract textual content.
This text will stroll via an extractive summarization course of, utilizing a easy phrase frequency strategy, applied in Python. Earlier than we start, notice that we’re not spending a lot power on information preprocessing, tokenization, normalization, and so forth. on this article (similar to last time), nor are we introducing any libraries that are in a position to simply and successfully carry out these duties. I wish to deal with presenting the textual content summarization steps, largely glossing over different essential ideas. I’m planning quite a few follow-ups to this piece, and we’ll add rising complexity to our NLP duties as we go.
Additionally, and for instance, since we’re doing a little minimal tokenization right here, out of necessity, you’ll get a really feel for when it’s being carried out, and doing so extra successfully can optionally be left as an train for the reader.
Let’s be clear about what we’re going to do right here:
- Take textual enter (a brief information article)
- Carry out minimal textual content preprocessing
- Create an information illustration
- Carry out summarization utilizing this information illustration
There are a selection of how of performing textual content summarization, as famous above, and we shall be utilizing a really fundamental extractive methodology to take action which is predicated on phrase frequencies inside the given article.
As we’re not leaning on libraries for nearly something, our imports are few:
from collections import Counter from string import punctuation from sklearn.feature_extraction.stop_words import ENGLISH_STOP_WORDS as stop_words
We want the punctuation
and stop_words
modules with a purpose to determine these after we are scoring our phrases and, in the end, sentences for his or her perceived significance, and we’ll deem neither punctuation nor cease phrases “essential” for this activity. Why so? Versus a language modeling activity the place these would unquestionably be helpful, or maybe a textual content classification activity, it ought to be apparent that together with steadily occurring cease phrases or repetitive punctuation would result in biasing in the direction of these tokens, offering no profit to us. There are all types of the explanation why we might wish to not exclude cease phrases (their arbitrary elimination ought to be prevented), however this doesn’t appear to be one in every of them.
Subsequent, we’d like some textual content to check our summarization approach on. I manually copied and pasted this one from CNN, however be happy to search out your personal:
# https://www.cnn.com/2019/11/26/politics/judiciary-committee-hearing/index.html textual content = """ The Home Judiciary Committee has invited President Donald Trump or his counsel to take part within the panel's first impeachment listening to subsequent week because the Home strikes one other step nearer to impeaching the President. The committee introduced that it will maintain a listening to December 4 on the "constitutional grounds for presidential impeachment," with a panel of knowledgeable witnesses testifying. Home Judiciary Chairman Jerry Nadler despatched a letter to Trump on Tuesday notifying him of the listening to and welcoming the President or his counsel to take part, together with asking questions of the witnesses. "I write to ask when you or your counsel plan to attend the listening to or make a request to query the witness panel," the New York Democrat wrote. Within the letter, Nadler mentioned the listening to would "function a chance to debate the historic and constitutional foundation of impeachment, in addition to the Framers' intent and understanding of phrases like 'excessive crimes and misdemeanors.' " "We anticipate to debate the constitutional framework via which the Home might analyze the proof gathered within the current inquiry," Nadler added. "We will even talk about whether or not your alleged actions warrant the Home's exercising its authority to undertake articles of impeachment." The Judiciary Committee listening to is the newest signal that Home Democrats are transferring ahead with impeachment proceedings in opposition to the President following the two-month investigation led by the Home Intelligence Committee into allegations that Trump pushed Ukraine to research his political rivals whereas a White Home assembly and $400 million in safety support have been withheld from Kiev. The listening to announcement comes because the Intelligence Committee plans to launch its report summarizing the findings of its investigation to the Home Judiciary Committee quickly after Congress returns from its Thanksgiving recess subsequent week. Democratic aides declined to say what extra hearings they'll schedule as a part of the impeachment proceedings. The Judiciary Committee is predicted to carry a number of hearings associated to impeachment, and the panel would debate and approve articles of impeachment earlier than a vote on the Home flooring. The aides mentioned the primary listening to was a "authorized listening to" that would come with some historical past of impeachment, in addition to evaluating the seriousness of the allegations and the proof in opposition to the President. Nadler requested Trump to reply by Sunday on whether or not the White Home wished to take part within the hearings, in addition to who would act because the President's counsel for the proceedings. The letter was copied to White Home Counsel Pat Cipollone. """
Did I say we weren’t tokenizing? Effectively, we’re. Poorly. However let’s not deal with that proper now. We’ll want 2 easy tokenizing features: one for tokenizing sentences into phrases, and one other for tokenizing paperwork into sentences:
def tokenizer(s): tokens = [] for phrase in s.cut up(' '): tokens.append(phrase.strip().decrease()) return tokens def sent_tokenizer(s): sents = [] for despatched in s.cut up('.'): sents.append(despatched.strip()) return sents
We want particular person phrases with a purpose to decide their relative frequency within the doc, and assign a corresponding rating; we’d like particular person sentences to subsequently sum the scores of every phrase inside with a purpose to decide sentence “significance.”
Word the we’re utilizing “significance” right here as a synonym for the relative phrase frequency within the doc; we’ll divide the variety of occurrences of every phrase by the variety of occurrences of the phrase which happens most within the doc. Does such excessive frequency equal real significance? It’s naive to imagine that it does, but it surely’s additionally the only solution to introduce the idea of textual content summarization. Excited by difficult our assumption of “significance” right here? Attempt one thing like TF-IDF or phrase embeddings as a substitute.
Okay, let’s tokenize:
tokens = tokenizer(textual content) sents = sent_tokenizer(textual content) print(tokens) print(sents)
['the', 'house', 'judiciary', 'committee', 'has', 'invited', 'president', 'donald', 'trump', 'or', 'his', 'counsel', 'to', 'participate', 'in', 'the', "panel's", 'first', 'impeachment', 'hearing', 'next', 'week', 'as', 'the', 'house', 'moves', 'another', 'step', 'closer', 'to', 'impeaching', 'the', 'president.', 'the', 'committee', 'announced', 'that', 'it', 'would', 'hold', 'a', 'hearing', 'december', '4', 'on', 'the', '"constitutional', 'grounds', 'for', ... 'the', 'white', 'house', 'wanted', 'to', 'participate', 'in', 'the', 'hearings,', 'as', 'well', 'as', 'who', 'would', 'act', 'as', 'the', "president's", 'counsel', 'for', 'the', 'proceedings.', 'the', 'letter', 'was', 'copied', 'to', 'white', 'house', 'counsel', 'pat', 'cipollone.'] ["The House Judiciary Committee has invited President Donald Trump or his counsel to participate in the panel's first impeachment hearing next week as the House moves another step closer to impeaching the President", 'The committee announced that it would hold a hearing December 4 on the "constitutional grounds for presidential impeachment," with a panel of expert witnesses testifying', 'House Judiciary Chairman Jerry Nadler sent a letter to Trump on Tuesday ... seriousness of the allegations and the evidence against the President', "Nadler asked Trump to respond by Sunday on whether the White House wanted to participate in the hearings, as well as who would act as the President's counsel for the proceedings", 'The letter was copied to White House Counsel Pat Cipollone', '']
Do not look too carefully if you’re following alongside at residence, or else you will notice the place our easy tokenization strategy fails. Shifting on…
Now we have to rely the occurrences of every phrase within the doc.
def count_words(tokens): word_counts = {} for token in tokens: if token not in stop_words and token not in punctuation: if token not in word_counts.keys(): word_counts[token] = 1 else: word_counts[token] += 1 return word_counts word_counts = count_words(tokens) word_counts
{'home': 10, 'judiciary': 5, 'committee': 7, 'invited': 1, 'president': 3, ... "president's": 1, 'proceedings.': 1, 'copied': 1, 'pat': 1, 'cipollone.': 1}
Our poor tokenizing reveals up once more within the last token above. Within the subsequent article, I am going to present you alternative tokenizers you’ll be able to drop in place to assist with this. Why not do that from the beginning? As I mentioned, I wish to deal with the textual content summarization steps.
Now that we’ve our phrase counts, we are able to construct a phrase frequency distribution:
def word_freq_distribution(word_counts): freq_dist = {} max_freq = max(word_counts.values()) for phrase in word_counts.keys(): freq_dist[word] = (word_counts[word]/max_freq) return freq_dist freq_dist = word_freq_distribution(word_counts) freq_dist
{'home': 1.0, 'judiciary': 0.5, 'committee': 0.7, 'invited': 0.1, 'president': 0.3, ... "president's": 0.1, 'proceedings.': 0.1, 'copied': 0.1, 'pat': 0.1, 'cipollone.': 0.1}
And there we go: we divided the incidence of every phrase by the frequency of probably the most occurring phrase to get our distribution.
Subsequent we wish to rating our sentences through the use of the frequency distribution we generated. That is merely summing up the scores of every phrase in a sentence and hanging on to the rating. Our perform takes a max_len
argument which units a most size to sentences that are to be thought-about to be used within the summarization. It ought to be comparatively simple to see that, given the best way we’re scoring our sentences, we might be biasing in the direction of lengthy sentences.
def score_sentences(sents, freq_dist, max_len=40): sent_scores = {} for despatched in sents: phrases = despatched.cut up(' ') for phrase in phrases: if phrase.decrease() in freq_dist.keys(): if len(phrases) < max_len: if despatched not in sent_scores.keys(): sent_scores[sent] = freq_dist[word.lower()] else: sent_scores[sent] += freq_dist[word.lower()] return sent_scores sent_scores = score_sentences(sents, freq_dist) sent_scores
{"The Home Judiciary Committee has invited President Donald Trump or his counsel to take part within the panel's first impeachment listening to subsequent week because the Home strikes one other step nearer to impeaching the President": 6.899999999999999, 'The committee introduced that it will maintain a listening to December 4 on the "constitutional grounds for presidential impeachment," with a panel of knowledgeable witnesses testifying': 2.8000000000000007, 'Home Judiciary Chairman Jerry Nadler despatched a letter to Trump on Tuesday notifying him of the listening to and welcoming the President or his counsel to take part, together with asking questions of the witnesses': 5.099999999999999, '"I write to ask when you or your counsel plan to attend the listening to or make a request to query the witness panel," the New York Democrat wrote': 2.5000000000000004, 'Within the letter, Nadler mentioned the listening to would "function a chance to debate the historic and constitutional foundation of impeachment, in addition to the Framers' intent and understanding of phrases like 'excessive crimes and misdemeanors': 3.300000000000001, '' "n"We anticipate to debate the constitutional framework via which the Home might analyze the proof gathered within the current inquiry," Nadler added': 2.7, '"We will even talk about whether or not your alleged actions warrant the Home's exercising its authority to undertake articles of impeachment': 1.6999999999999997, 'The listening to announcement comes because the Intelligence Committee plans to launch its report summarizing the findings of its investigation to the Home Judiciary Committee quickly after Congress returns from its Thanksgiving recess subsequent week': 5.399999999999999, 'Democratic aides declined to say what extra hearings they'll schedule as a part of the impeachment proceedings': 1.3, 'The Judiciary Committee is predicted to carry a number of hearings associated to impeachment, and the panel would debate and approve articles of impeachment earlier than a vote on the Home flooring': 4.300000000000001, 'The aides mentioned the primary listening to was a "authorized listening to" that would come with some historical past of impeachment, in addition to evaluating the seriousness of the allegations and the proof in opposition to the President': 2.8000000000000007, "Nadler requested Trump to reply by Sunday on whether or not the White Home wished to take part within the hearings, in addition to who would act because the President's counsel for the proceedings": 3.5000000000000004, 'The letter was copied to White Home Counsel Pat Cipollone': 2.2}
Now that we’ve scored our sentences for his or her significance, all that is left to do is choose (i.e. extract, as in “extractive summarization”) the highest ok sentences to signify the abstract of the article. This perform will take the sentence scores we generated above in addition to a worth for the highest ok highest scoring sentences to sue for summarization. It should return a string abstract of the concatenated high sentences, in addition to the sentence scores of the sentences used within the summarization.
def summarize(sent_scores, ok): top_sents = Counter(sent_scores) abstract = '' scores = [] high = top_sents.most_common(ok) for t in high: abstract += t[0].strip()+'. ' scores.append((t[1], t[0])) return abstract[:-1], scores
Let’s use the perform to generate the abstract.
abstract, summary_sent_scores = summarize(sent_scores, 3) print(abstract)
The Home Judiciary Committee has invited President Donald Trump or his counsel to take part within the panel's first impeachment listening to subsequent week as the Home strikes one other step nearer to impeaching the President. The listening to announcement comes because the Intelligence Committee plans to launch its report summarizing the findings of its investigation to the Home Judiciary Committee quickly after Congress returns from its Thanksgiving recess subsequent week. Home Judiciary Chairman Jerry Nadler despatched a letter to Trump on Tuesday notifying him of the listening to and welcoming the President or his counsel to take part, together with asking questions of the witnesses.
And let’s take a look at the abstract sentence scores for good measure.
for rating in summary_sent_scores: print(rating[0], '->', rating[1], 'n')
6.899999999999999 -> The Home Judiciary Committee has invited President Donald Trump or his counsel to take part within the panel's first impeachment listening to subsequent week because the Home strikes one other step nearer to impeaching the President 5.399999999999999 -> The listening to announcement comes because the Intelligence Committee plans to launch its report summarizing the findings of its investigation to the Home Judiciary Committee quickly after Congress returns from its Thanksgiving recess subsequent week 5.099999999999999 -> Home Judiciary Chairman Jerry Nadler despatched a letter to Trump on Tuesday notifying him of the listening to and welcoming the President or his counsel to take part, together with asking questions of the witnesses
The abstract appears affordable at a fast cross, given the textual content of the article. Check out this straightforward methodology on another textual content for additional proof.
The following summarization article will construct on this straightforward methodology in a number of key methods, particularly:
- correct tokenization approaches
- enchancment to our baseline strategy, utilizing TF-IDF weighting as a substitute of easy phrase frequency
- use of an precise dataset for our summarization
- analysis of our outcomes
See you subsequent time.
Matthew Mayo (@mattmayo13) is a Information Scientist and the Editor-in-Chief of KDnuggets, the seminal on-line Information Science and Machine Studying useful resource. His pursuits lie in pure language processing, algorithm design and optimization, unsupervised studying, neural networks, and automatic approaches to machine studying. Matthew holds a Grasp’s diploma in laptop science and a graduate diploma in information mining. He could be reached at editor1 at kdnuggets[dot]com.
[ad_2]
Source link